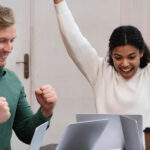
Impact of Fun at Work Activities on Employee Engagement
4th June 2024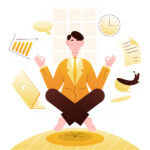
Mindfulness and Meditation for Busy Professionals
11th June 2024Flutter GetX is a state management library that is lightweight, quick, and stable. It is a sophisticated microframework that allows us to create routing, manage states, and execute dependency injection.
But when developing a Flutter application, we must consider which state management to use.
Many state management libraries are in Flutter, such as BLoC, MobX, Provider, Redux, etc.
Getx is one among them!
This article examines Flutter GetX, its installation, features, and advantages.
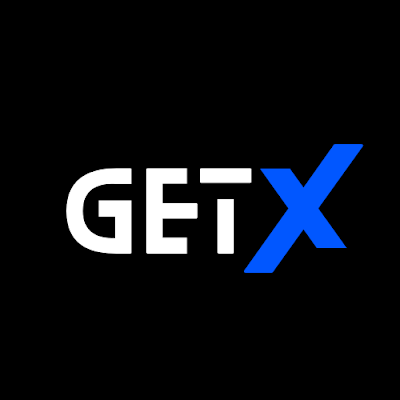
What is GetX in Flutter state management?
When using Flutter, you create your application using widgets that organize themselves into tree-like structures, each with child widgets that have other child widgets. Sometimes a change in one widget must cause a change in another.
Changes might be passed on through several generations of widgets to get the desired result. But it would help if you change the widgets in the same tree with a new hierarchy.
You must add a new widget to share a common parent widget with other widgets in the tree. It could become a nightmare when the widget tree is complicated, or you need to make fewer modifications.
Here comes Flutter GetX!
GetX is a powerful and lightweight Flutter package that allows developers to handle complicated route management, state management, and dependency injection. GetX in Flutter simplifies things like never before in your Flutter application.
It is built around three fundamental principles: performance, productivity, and organization.
Three principles of Flutter GetX
GetX is based on three basic principles:
Performance
GetX’s primary focus is on performance and resource utilization. Flutter Getx is the best solution among the other state management strategies because it does not use Streams or ChangeNotifier.
For memory usage optimization on most platforms, we must release the controller once it has finished being used. Flutter GetX does not perform this by default. If required, we can explicitly set the controller always to be true.
Productivity
GetX’s syntax is simple. It saves developers time and speeds up the program using no additional assets. It just uses the currently required assets, and after the task is completed, the assets are released naturally. It will not be simply valuable if all assets are stored in memory.
Organization
GetX code is organized into View, Logic, Route, and Dependency Injection components. So we don’t need any more context to navigate between screens. We can browse the screen without using the particular case; thus, we are not reliant on the widget tree.
The three pillars of GetX
State Management
In GetX, there are two state managers. The first is a simple state manager that uses the GetBuilder function. In contrast, the second is a reactive state manager that uses Getx or Obx.
- Not necessary to create a StreamController
- There is no requirement to create a Stream Builder.
- No requirement to create a class for each state
- There is no need to create an initial value
Make the variable observable in the following way:
1 var name = ‘Foo’.obs;
2 Obx(() => Text(“${controller.name}”));
Wherever the variable is used, all widgets will be updated.
Route Management
To create Widgets such as Snackbars, Bottom Sheets, Dialogues, and so on. Then we can include GetX in it because GetX can compose these widgets without using context.
GetX allows you to navigate to any screen with little or no coding.
Simply take these essential steps:
Add “Get” before the name of your app.
1 GetMyApp( // Before: MaterialApp
2
3 home: MyHome(),
To navigate to a new screen
1 Get.to(NextScreen());
Dependency Management
Flutter GetX uses controllers to provide a simple yet powerful solution for dependency management. It can be accessed from the view with a single line of code, without the need for an inherited widget or context.
Normally, you would instantiate a class within a class, but when you use GetX, you are instantiating the Get instance, which will be available across your program.
What is the use of GetX in Flutter?
Flutter GetX, a state manager for Flutter applications, provides various benefits, making it a popular choice among developers.
Here are some of the main benefits of using GetX in Flutter state management:
Simplicity and productivity
Flutter GetX has a straightforward and intuitive API that makes it simple to understand and use. It supports a more concise and understandable codebase by reducing boilerplate code. This ease of use increases productivity because developers can concentrate on designing features rather than dealing with sophisticated state management algorithms.
Reactive programming
Flutter GetX uses reactive programming techniques, allowing developers to build reactive state variables that automatically update the user interface when they change. This reactive technique makes synchronizing state changes with the user interface easier, resulting in a more responsive and interactive user experience.
Lightweight
Flutter GetX is intended to be light and efficient. It reduces the number of unwanted rebuilds by updating just the components dependent on the altered state. Even in complicated applications with many widgets, this optimization results in faster rendering and increased performance.
Dependency injection
Flutter GetX features a dependency injection method that simplifies managing dependencies within your application. You can use dependency injection to separate distinct sections of your codebase, promote modularity, and make testing and maintenance easier.
Powerful routing system
Flutter GetX provides a robust and adaptable routing system. It enables you to create named routes, transfer parameters between displays, and handle complex navigation scenarios easily. The routing system simplifies screen transition management and improves code organization within your app.
Community support
Flutter GetX has a developing and active developer community that contributes to its growth, provides help, and shares resources. This active community guarantees access to documentation, tutorials, and sample projects, making it easy to get started and troubleshoot any emerging issues.
Integration with the Flutter ecosystem
Flutter GetX works in tandem with other Flutter libraries and packages. It can be used with popular libraries such as Flutter Material or Cupertino widgets and other state management solutions if necessary. This adaptability enables you to use several libraries’ benefits and tailor your app architecture to your individual needs.
These benefits make Flutter GetX an appealing alternative for state management in Flutter applications, offering developers a robust and fast solution to handle the state of their app while keeping simplicity and efficiency.
GetX installation
Go to the GetX install page on pub.dev and copy the line of code to add in your pubspec.yaml file to install GetX. The current version of GetX is 4.6.1 as of the time of writing. As a result, we shall paraphrase:
1 get: ^4.6.5 //YAML
2
3 get_storage: ^2.0.3 //YAML
Then copy and paste it into our pubspec.yml file’s dependencies section. Get should be installed immediately when you save the file. Alternatively, you can run the command directly on your terminal.
1 flutter pub get //YAML
Your pubspec.yaml file’s dependencies section should look like this:
1 dependencies:
2 flutter:
3 sdk: flutter
4 get: ^4.6.5
GetxController
Controllers are classes that house all of our business logic. All variables and methods are stored in this location and can be accessed from the view.
While we could write a simple class to accomplish this, GetX offers a class named GetxController that extends DisposableInterface.
This means that when the widgets that use our controller are removed from the navigation stack, our controller will be destroyed from memory. We don’t need to dispose of anything manually, and memory usage is lowered, resulting in a good performance.
GetxController includes onInit() and onClose() methods that basically replace the StatefulWidget’s initState() and dispose() methods. This allows us to avoid using StatefulWidget entirely and create well-organised code.
1 class Controller extends GetxController {
2
3 @override
4
5 fetchApi();
6
7 }
8
9 @override
10
11 showIntroDialog();
12
13 }
14
15 @override
16
17 closeStream();
18
19 }
20
21 }
GetBuilder
GetBuilder can be wrapped around any widget to make it communicate with the controller’s methods and variables. We’ll be able to call functions, monitor state changes, and so on.
1 GetBuilder<Controller>( // specify type as Controller
2 init: Controller(), //initialize with the Controller
3 builder: (value) => Text(
4 '${value.counter}', // value is an instance of Controller.
5 ),
6 ),
7
8 GetBuilder<Controller>( // no need to initialize Controller ever again, just mention the type
9 builder: (value) => Text(
10 '${value.counter}', // counter is updated when increment() is called
11 ),
12 ),
read more about flutter click here
visit us xpertlab