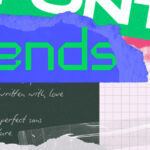
Top Font Trends of 2025: Human, Futuristic and Bold
4th February 2025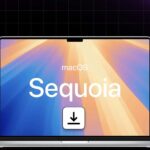
macOS Sequoia 15.3 Update Everything You Need to Know!
10th February 2025in this tutorial, we’ll be exploring the topic of higher order functions in React. Once we’ve covered the basics, we’ll delve deeper into the world of higher order components and examine how they can be used in real-world scenarios. Drawing from my own experience developing React applications, I’ll provide concrete examples to help you gain a thorough understanding of these concepts. So, let’s roll up our sleeves and get started on taking your React development skills to the next level!
Table of content
- What are Higher Order Functions in React Js and how to use them?
- What is a Higher Order Component?
- Structure and basic syntax of HOCs
- Real world use case
Higher Order Function:
In React, a higher-order function is a function that takes one or more functions as arguments and returns a new function that uses or composes those functions.

The best thing about using higher-order functions is that we can put together small functions to make bigger, more complicated functions. By using this method, we can avoid errors in our code and make it simpler to understand.
Consider this Use Case:
Imagine we want to create a function that multiplies two numbers together. Instead of making a function that takes in both numbers at once, we can create a higher-order function that takes in one number and returns a new function that asks for the second number. That way, we can reuse the function to multiply any two numbers, just by calling the higher-order function with the first number, and then using the returned function to get the second number and calculate the product. This saves us time and makes our code more flexible.
function Calculator(num) {
return function(x) {
return x * num;
}
}
const multiplyByTwo = multiplyBy(2);
const multiplyByFive = multiplyBy(5);
console.log(multiplyByTwo(4)); // Output: 8
console.log(multiplyByFive(10)); // Output: 50
.forEach()
This iterates over every element in an array with the same code, but does not change or mutate the array, and it returns undefined..map()
This method transforms an array by applying a function to all of its elements, and then building a new array from the returned values.
Let’s talk about Higher Order Components now:

A higher-order component (HOC) is a way to reuse logic in React components. It’s a component that takes in other dumb components as inputs and returns a new, improved component. HOCs work similarly to higher-order functions, which take in functions as inputs and produce a new function.
HOCs are often used to create components that share certain behaviors in a way that’s different from the usual way of connecting components through state and props. They help make our code more modular and easier to reuse.
Let’s understand this with an example:
import React from 'react';
const withLogger = (WrappedComponent) => {
return class extends React.Component {
componentDidMount() {
console.log('Component is mounted');
}
render() {
return <WrappedComponent {...this.props} />;
}
}
}
const MyComponent = () => {
return <div>Hello, World!</div>;
};
const EnhancedComponent = withLogger(MyComponent);
export default EnhancedComponent;
Suppose, we have a higher-order component called withLogger
that takes a component (WrappedComponent
) as an argument and returns a new component with additional logging functionality. The returned component logs a message to the console when it’s mounted, and then renders the WrappedComponent
with its props.
We then create a simple component called MyComponent
, and use the withLogger
higher-order component to create a new component called EnhancedComponent
. EnhancedComponent
is now a component that logs a message to the console when it’s mounted and also renders the MyComponent
component.
Structure of HOCs:
Here’s an example of how a HOC might look in React:
import React from 'react';
// Take in a component as argument WrappedComponent
const higherOrderComponent = (WrappedComponent) => {
// And return another component
class HOC extends React.Component {
render() {
return <WrappedComponent />;
}
}
return HOC;
};
We can observe that the HigherOrderComponent
function accepts a component (WrappedComponent
) as its argument and returns a new component that contains it. This approach enables us to extract and reuse the logic of a particular component by creating a HOC based on it. Once we’ve created the HOC, we can use it in any part of our application where that logic is needed.
When working with higher-order components (HOCs) in React, there are some basic syntax rules to follow.
First, it’s important to remember that a HOC is a type of component. It takes in another component as an argument, and then returns a new, enhanced component that can render the original component that was passed to it.
To structure a HOC in React, you can think of it as a higher-order function. Just like a higher-order function takes in a function as an argument and returns a new function, a HOC takes in a component as an argument and returns a new, enhanced component.
Real World use case of HOC that I have used in couple of my projects:
For instance, let’s say you have a few components in your application that require users to be authenticated before they can access them. You could create a HOC that wraps each of these components, and adds the authentication logic to them
import React from 'react';
const withAuth = (WrappedComponent) => {
return class extends React.Component {
constructor(props) {
super(props);
this.state = {
isAuthenticated: false
}
}
componentDidMount() {
// check if user is authenticated
// if so, update state
if (/* user is authenticated */) {
this.setState({
isAuthenticated: true
});
}
}
render() {
if (this.state.isAuthenticated) {
return <WrappedComponent {...this.props} />;
} else {
return <div>You must be logged in to view this page.</div>;
}
}
}
}
To use this HOC, you would simply wrap any components that require authentication with it:
import React from 'react';
import withAuth from './withAuth';
const Profile = (props) => {
return <div>Welcome to your profile, {props.username}!</div>;
}
export default withAuth(Profile);
contact us : xpertlab
similar blog : reactjs