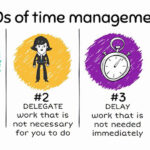
How to Prioritize Tasks Effectively: A Developer’s Guide to Productivity
28th February 2025React Hooks are functions that enable functional components to use React state and lifecycle features. They eliminate the need for class components, making code cleaner and easier to maintain
As you may know, React is a open-source library used to build user interfaces in a simpler and more efficient way than tools that came before (vanilla JS and jQuery mainly). It was developed by Meta (aka Facebook) and released to the public in 2013.
Hooks were a feature introduced years later in 2016 (in React’s 16.8 version). Just to have an idea of what hooks are for and why are they improvement over what was done before, let’s view an example of “pre-hooks” code against some modern React “post-hooks” code.
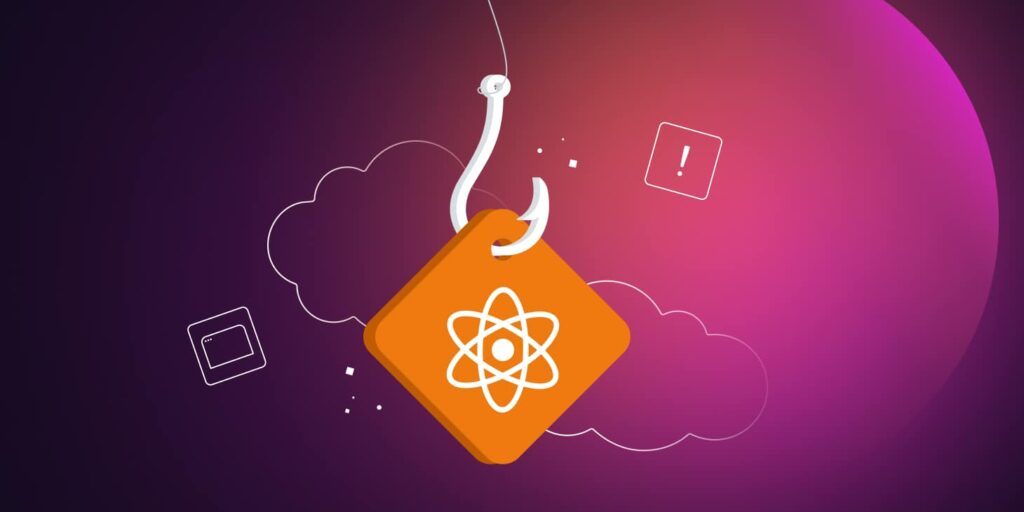
Types of React Hooks
1. State Hooks
The useState hook is an alternative to the useReducer hook that is preferred when we require the basic update. useState Hooks are used to add the state variables in the components. For using the useState hook we have to import it in the component.
import React, { useState } from "react";
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() =>
setCount(count + 1)}>Increment</button>
</div>
);
}
export default Counter;
- useState initializes the count variable with 0 and provides a method setCount to update it.
- Clicking the button updates the state using the setCount function.
2. Context Hooks
The useContext hook in React allows components to consume values from the React context. React’s context API is primarily designed to pass data down the component tree without manually passing props at every level. useContext is a part of React’s hooks system, introduced in React 16.8, that enables functional components to access context values.
import React, { createContext, useContext } from "react";
const Contexts = createContext("light");
function Theme() {
const theme = useContext(Contexts);
return <h1>Theme: {theme}</h1>;
}
export default function App() {
return (
<Contexts.Provider value="dark">
<Theme />
</Contexts.Provider>
);
}
- The Theme Context provides a value (“dark”) accessible via useContext in DisplayTheme.
- This eliminates the need to pass props manually down the component tree.
3. Ref Hooks
The useRef Hook is a built-in React Hook that returns a mutable reference object (ref) that persists across renders. Unlike state variables, updating a ref does not trigger a component re-render.
import React, { useRef } from "react";
function Focus() {
const input = useRef();
const focus = () => input.current.focus();
return (
<div>
<input ref={input} type="text" />
<button onClick={focus}>Focus</button>
</div>
);
}
export default Focus;
- useRef stores a reference to the input element, allowing the focus function to programmatically set focus on it.
- Updating inputRef does not cause the component to re-render.
4. Effect Hooks
React useEffect hook handles the effects of the dependency array. The useEffect
Hook allows us to perform side effects on the components. fetching data, directly updating the DOM and timers are some side effects. It is called every time any state if the dependency array is modified or updated.
import React, { useEffect, useState } from "react";
function Timer() {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() =>
setSeconds((s) => s + 1), 1000);
return () => clearInterval(interval);
}, []);
return <h1>Time: {seconds}s</h1>;
}
export default Timer;
- The useEffect hook starts an interval to update the seconds state every second.
- The cleanup function ensures the interval is cleared when the component unmounts.
5. Performance Hooks
The useMemo Hook is a built-in React Hook that helps optimize performance by memoizing the result of a computation and reusing it unless its dependencies change. This prevents expensive computations from being re-executed unnecessarily during component re-renders.
Syntax
const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]);
The useCallback Hook is a built-in React Hook that memoizes a callback function, preventing it from being recreated on every render unless its dependencies change. This is useful for optimizing performance, especially when passing functions as props to child components.
Syntax
const memoizedCallback = useCallback(() => { // Function logic }, [dependencies]);
import React, { useMemo } from "react";
function ExpCal({ num }) {
const compute = useMemo(() => num * 2, [num]);
return <h1>Result: {compute}</h1>;
}
- useMemo caches the computed value (num * 2), recalculating it only when num changes.
- This prevents unnecessary calculations on every render.
6. Resource Hooks
These include useFetch or custom hooks for fetching and managing external resources.
function useFetch(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then((response) => response.json())
.then((data) => setData(data));
}, [url]);
return data;
}
- useFetch is a custom hook for fetching data from a given URL.
- It uses useEffect to fetch data when the URL changes and updates the data state.
contact us: xpertlab
similar blog: click here