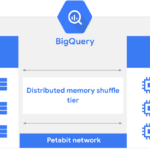
What is Google BigQuery and How Does it Work? – The Ultimate Guide
12th March 2025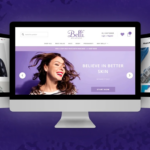
7 Beautiful Websites To Inspire Your Own
18th March 2025What is GraphQL?
GraphQL is a query language for APIs and a runtime for executing those queries against your data. It allows clients to request only the needed data, reducing over-fetching and under-fetching issues common in REST APIs. Developed by Facebook, GraphQL enables more flexible and efficient data fetching by allowing clients to define the shape of the response.
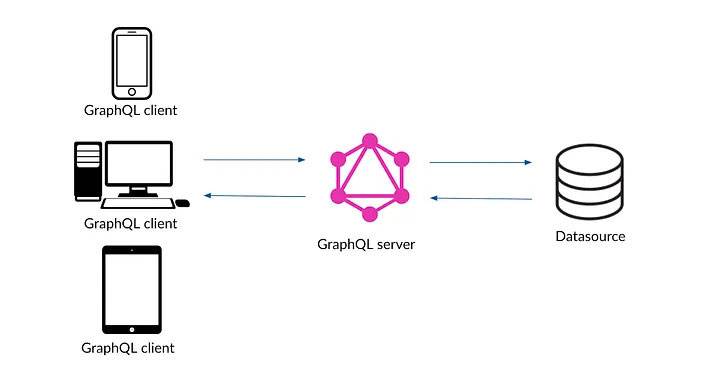
Key Features of GraphQL:
- Declarative Data Fetching: Clients specify the exact data they need.
- Single Endpoint: Unlike REST, which has multiple endpoints, GraphQL uses a single endpoint.
- Strongly Typed Schema: Defines the structure of the API using types.
- Efficient Data Loading: Supports nested and related data fetching in a single request.
Limitations of GraphQL:
- Complex Caching – Unlike REST, where caching is straightforward with HTTP methods, GraphQL requires custom caching mechanisms.
- Overhead in Small Requests – Query parsing and execution can introduce more processing time compared to simple REST endpoints.
- Security Challenges – Deeply nested queries can lead to performance bottlenecks (e.g., infinite loops or DDoS attacks).
- Complex Authorization & Authentication – Requires implementing field-level access control, which can be more complex than REST.
- File Uploading Limitations – GraphQL does not natively support file uploads; workarounds like using REST endpoints or
graphql-upload
are needed. - Schema Complexity – Maintaining a well-structured GraphQL schema can become difficult as the API scales.
- Learning Curve – Requires developers to understand resolvers, schema design, and query optimization.
Feature | REST API | GraphQL API |
Data Fetching | Multiple endpoints for different resources | Single endpoint (/graphql ) with flexible queries |
Over-fetching & Under-fetching | Can return unnecessary data or require multiple requests | Clients request only the needed fields |
Schema | No strict schema enforcement | Strongly typed schema with SDL (Schema Definition Language) |
Efficiency | May require multiple requests to fetch related data | Fetches related data in a single request |
Caching | Easy with HTTP caching (GET requests) | Requires custom caching logic |
Performance | Efficient for simple data retrieval | May add overhead due to query parsing |
File Uploads | Supported via multipart form-data | Needs workarounds (e.g., graphql-upload ) |
Versioning | Uses versioned URLs (/v1 , /v2 ) | Evolving schema without breaking changes |
Error Handling | Uses HTTP status codes (400 , 404 , etc.) | Returns structured error responses |
Learning Curve | Easier to adopt | Requires learning resolvers, schema, and query optimization |
Create the GraphQL API
Create a server.js
file in your project and write the following code:
const express = require(‘express’);
const { graphqlHTTP } = require(‘express-graphql’);
const { buildSchema } = require(‘graphql’);
// Define the GraphQL schema
const schema = buildSchema(`
type Query {
hello: String
user(id: ID!): User
}
type User {
id: ID!
name: String!
email: String!
}
type Mutation {
createUser(name: String!, email: String!): User
}
`);
// Root provides a resolver for each API endpoint
const root = {
hello: () => ‘Hello, world!’,
user: ({ id }) => users.find(user => user.id === id),
createUser: ({ name, email }) => {
const newUser = { id: String(users.length + 1), name, email };
users.push(newUser);
return newUser;
}
};
// In-memory data storage for users
let users = [
{ id: “1”, name: “Alice”, email: “alice@example.com” },
{ id: “2”, name: “Bob”, email: “bob@example.com” }
];
// Set up the Express server
const app = express();
app.use(‘/graphql’, graphqlHTTP({
schema: schema,
rootValue: root, // Root object with resolvers
graphiql: true // Enable GraphiQL interface
}));
app.listen(4000, () => console.log(‘Server running at http://localhost:4000/graphql’));
Start the Server
node server.js
Request Parameaters:
{
user(id: “1”) {
name
email
}
}
Response:
{
”data”: {
”user”: {
”name”: “Alice”,
”email”: “alice@example.com”
}
}
}
Similar Content Click
For more information contact XpertLab